How to Create an Array:-
Every Array in java is an object.
Hence we can create an array by using new operator or keyword.
example:-
int [ ] a=new int [3] ;

Rules For Creating 1-D Array:-
1.At the time of Array creation compulsory, we should specify the size otherwise, we will get compile time error.
example:-
int [ ] x= new [ ]; (invalid)
int [ ] x= new [ 6 ]; (valid)
2.It is legal to have an Array with size 0 in java.
example:-
int [ ] x= new [ 0 ]; (valid)
3.If we are trying to specify array size with negative int value then we will get RunTime Exception saying
" NegativeArraySizeException".
example:-
int [ ] x= new [-3 ]; (invalid)
RuntimeException:- " NegativeArraySizeException".
But we won't get any Compile Time Error.The code will compile fine.
4.To specify Array size allowed data types are the byte, short, char, int.
If we are trying to specify any other than that then we will get compile time array.
example:-
int [ ] x= new [ 10]; (valid)
int [ ] x= new [ 'a']; (valid)
byte c=20
int [ ] x= new [ c]; (valid)
short s=30;
int [ ] x= new [ s]; (valid)
int [ ] x= new [ 10 L]; (invalid)
Compile Time Error:- Possible loss of precision
found: -long
required: -int
5.The maximum Array size in java is 2147483647 which is nothing but the maximum value of int data type.
int [ ] x= new [ 2147483647]; (valid)
int [ ] x= new [ 2147483647]; (invalid)
Compile Time Error:Integer Number Too Large
Note : In the 1st example of rule 5 we may get Runtime Exception if sufficient heap memory is not available.
2-D Array or Multidimensional Array
To create a 2-D array or multidimensional Array we have to specify the base size of the Array.Because we are using new operator for allocating memory to an array so Compulsory we should provide size at least base.For Array, creation java follows Array of Array Approach.
example 1:
If we are not Specifying the base size then we will get Compile time Error.
x[ 0] =new int [2];
x[1] =new int [3];
Memory representation of above example:
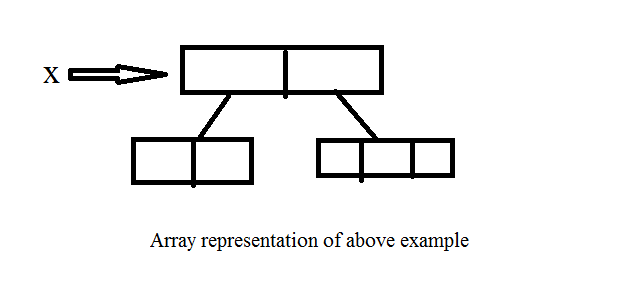
int [ ] [ ][ ] x =new int [ 3] [ ] [ ]; (valid)
In the above example, we provided the base value 3. Rest of we kept empty which is valid in java.
we won't get any compile time error or runtime exception;
example 3:
int [ ] [ ] x = new int [ ] [ 2]; (invalid)
In the above example we did' provide any base value, means base index is empty so it is wrong in java.
Hence we will get Compile time Error.
Que:
which of Array Declaration are valid?
int [ ] a =new int [ ];
int [ ] a =new int [ 3];
int [ ] [ ] a =new int [ ] [ ];
int [ ] [ ]a =new int [ 2] [ ];
int [ ] [ ]a =new int [ ] [ 4];
int [ ] [ ]a =new int [ 2] [3 ];
int [ ] [ ] [ ]a =new int [ 2] [ 4] [ 5];
int [ ] [ ] [ ]a =new int [ ] [ 1] [2 ];
int [ ] [ ] [ ]a =new int [ 2] [ ] [3 ];
Now scroll down for check your answers;
Ans
int [ ] a =new int [ ]; (invalid)
int [ ] a =new int [ 3]; (valid)
int [ ] [ ] a =new int [ ] [ ]; (invalid)
int [ ] [ ]a =new int [ 2] [ ]; (valid)
int [ ] [ ]a =new int [ ] [ 4]; (invalid)
int [ ] [ ]a =new int [ 2] [3 ]; (valid)
int [ ] [ ] [ ]a =new int [ 2] [ 4] [ 5]; (valid)
int [ ] [ ] [ ]a =new int [ 2] [ 4] [ 3]; (valid)
int [ ] [ ] [ ]a =new int [ ] [ 1] [2 ]; (invalid)
int [ ] [ ] [ ]a =new int [ 2] [ ] [3 ]; (valid)
No comments:
Post a Comment
Be the first to comment!
Don't just read and walk away, Your Feedback Is Always Appreciated. I will try to reply to your queries as soon as time allows.
Note:
1. If your question is unrelated to this article, please use our Facebook Page.
2. Please always make use of your name in the comment box instead of anonymous so that i can respond to you through your name and don't make use of Names such as "Admin" or "ADMIN" if you want your Comment to be published.
Regards,
JavaByChetan
Back To Home